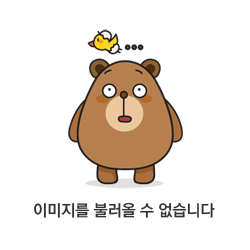
The goals
💪🏻How NoSQL Database Are Designed & Work
✌🏻Installing & Setting Up MongoDB
👍🏻Querying Data (CRUD Operations)
The Idea Behind NoSQL Database Systems
Store data without focusing on a strict schemas / data structures or relationships across multiple tables
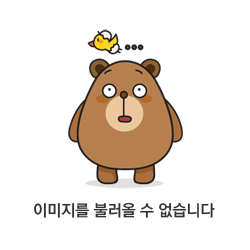
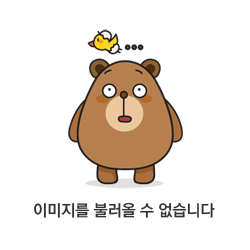
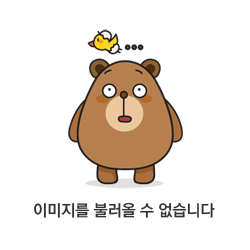
Let's try MongoDB
So let's try with mongoDB
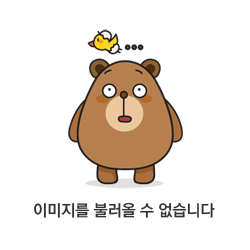
We need to download it first.
In my case, I already have downloaded MongoDB, and MongoDB Compass.
So I will only download MongoDB Shell.
Or we can use embedded MongoDB Shell on MongoDB Compass.
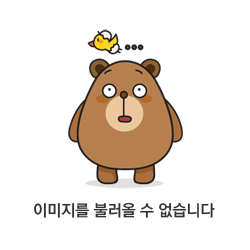
I run the code to use mongo shell
But I have this error....
So since I am capturing and writing this blog to organize and review once again.
I found out that they asked me to make a directory for mongosh
So I made folder and moved the files I got when I downloaded mongosh
and it worked!!!! yay!!!!!
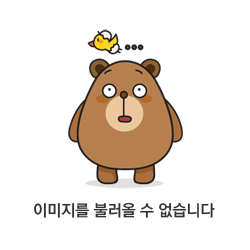
So now we can see 'test' line and we are available to write mongodb commands here.
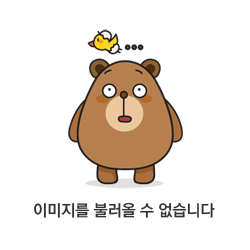
Connected well!! yay

So, now we define the data and our MongoDB automatically gives our data Id.

I made some typo here....
I should update laterㅠㅠ

So here we only got the name except address since we chose to see name

In general, by typing zero for a field, it's excluded
And typing 1 makes it included.
db.restaurants.find({}, {name: 1, _id: 0}
So the first parameter value allows us to define conditions to control which documents are fetched.
The second parameter value allows us to control which fields are shown for the fetched documents.

Now we can fetch only one data and this is not in array since it's only one.
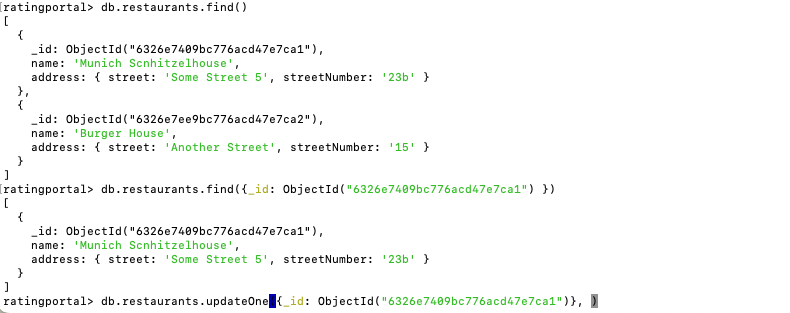
And here we can choose the area we want to update.

We can just put the area like this or we can put also nested data.

So now we have confirmation message.
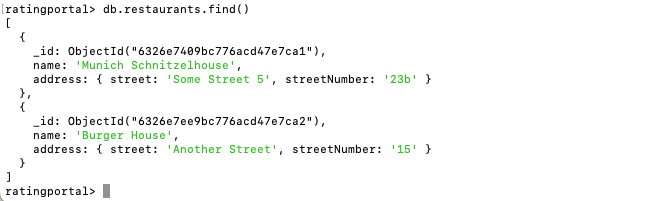
Now I fixed my name typo....hehe😅
Let's try again for nested data.
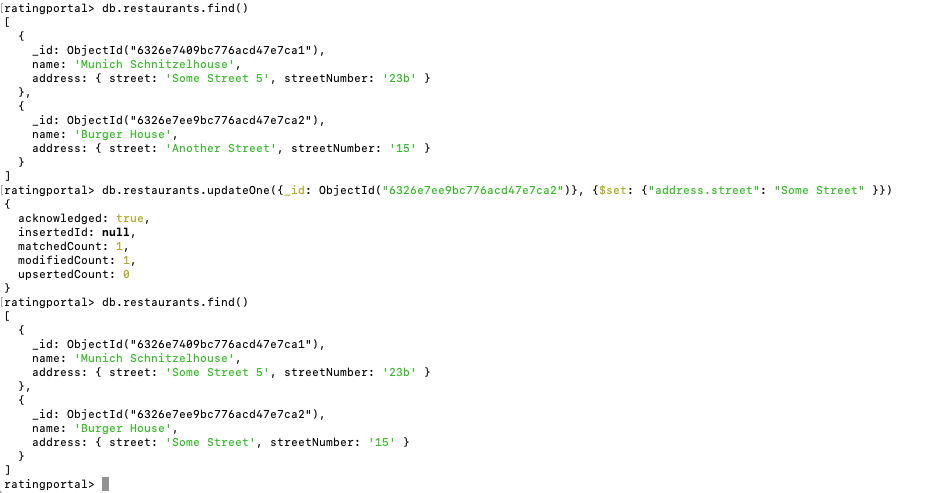
Yeah I changed the street name:)
I used only 'updateOne' in this example, but we can also use 'updateMany'
db.collection.updateMany()
Definition
db.collection.updateMany(filter, update, options)
Examples
Update Multiple Documents
The restaurant collection contains the following documents:
{ "_id" : 1, "name" : "Central Perk Cafe", "violations" : 3 }
{ "_id" : 2, "name" : "Rock A Feller Bar and Grill", "violations" : 2 }
{ "_id" : 3, "name" : "Empire State Sub", "violations" : 5 }
{ "_id" : 4, "name" : "Pizza Rat's Pizzaria", "violations" : 8 }
try {
db.restaurant.updateMany(
{ violations: { $gt: 4 } },
{ $set: { "Review" : true } }
);
} catch (e) {
print(e);
}
The operation returns:
{ "acknowledged" : true, "matchedCount" : 2, "modifiedCount" : 2 }
The collection now contains the following documents:
{ "_id" : 1, "name" : "Central Perk Cafe", "violations" : 3 }
{ "_id" : 2, "name" : "Rock A Feller Bar and Grill", "violations" : 2 }
{ "_id" : 3, "name" : "Empire State Sub", "violations" : 5, "Review" : true }
{ "_id" : 4, "name" : "Pizza Rat's Pizzaria", "violations" : 8, "Review" : true }
If no matches were found, the operation instead returns:
{ "acknowledged" : true, "matchedCount" : 0, "modifiedCount" : 0 }
Setting upsert: true would insert a document if no match was found.
db.collection.deleteOne()
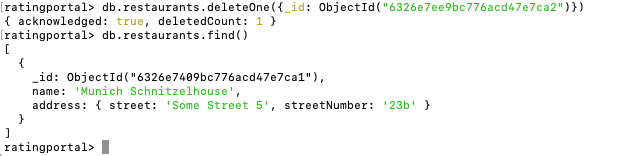
db.collection.deleteMany()
Examples
Delete Multiple Documents
The orders collection has documents with the following structure:
{
_id: ObjectId("563237a41a4d68582c2509da"),
stock: "Brent Crude Futures",
qty: 250,
type: "buy-limit",
limit: 48.90,
creationts: ISODate("2015-11-01T12:30:15Z"),
expiryts: ISODate("2015-11-01T12:35:15Z"),
client: "Crude Traders Inc."
}
The following operation deletes all documents where client : "Crude Traders Inc.":
try {
db.orders.deleteMany( { "client" : "Crude Traders Inc." } );
} catch (e) {
print (e);
}
The operation returns:
{ "acknowledged" : true, "deletedCount" : 10 }
The following operation deletes all documents where stock : "Brent Crude Futures" and limit is greater than 48.88:
try {
db.orders.deleteMany( { "stock" : "Brent Crude Futures", "limit" : { $gt : 48.88 } } );
} catch (e) {
print (e);
}
The operation returns:
{ "acknowledged" : true, "deletedCount" : 8 }
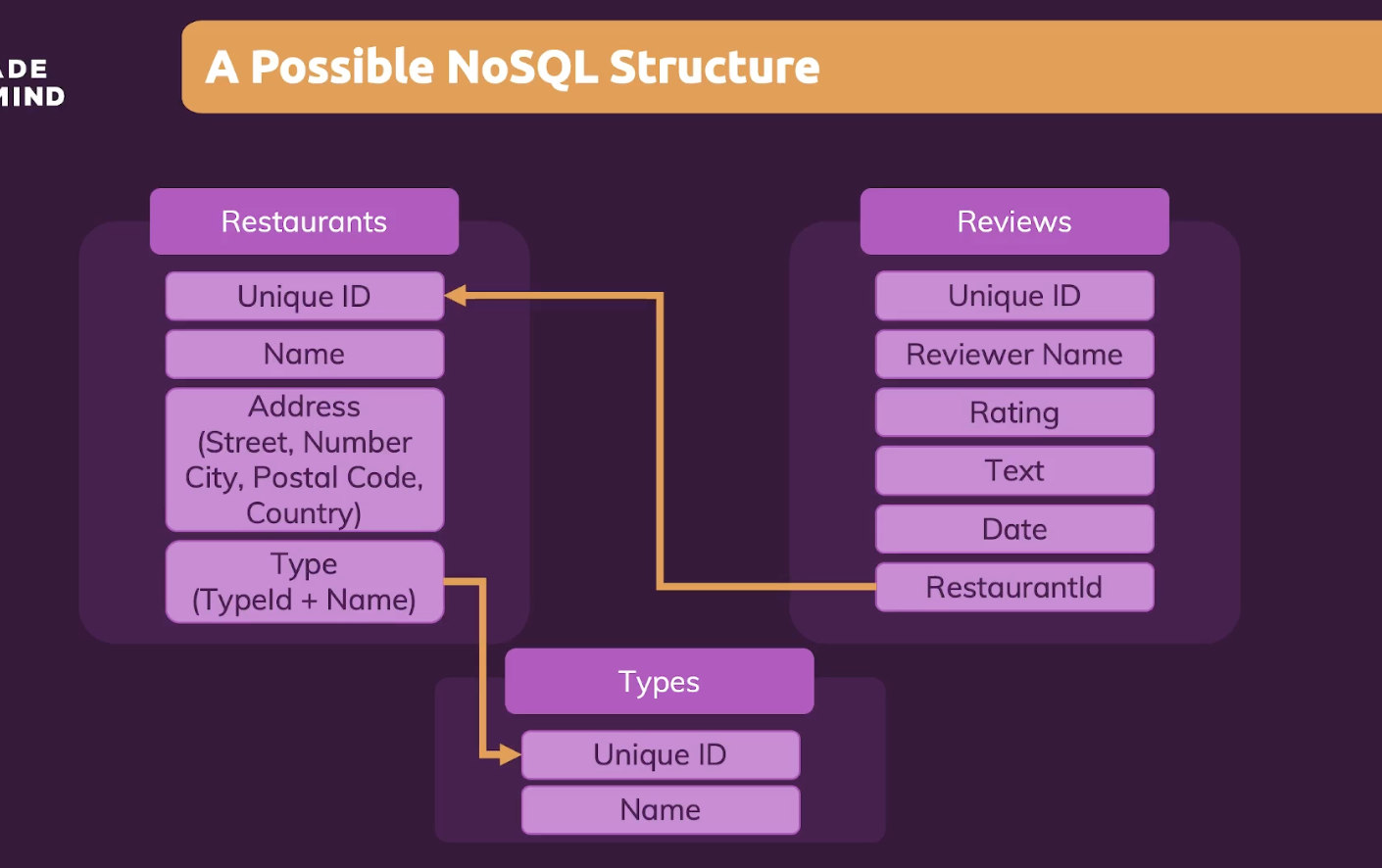
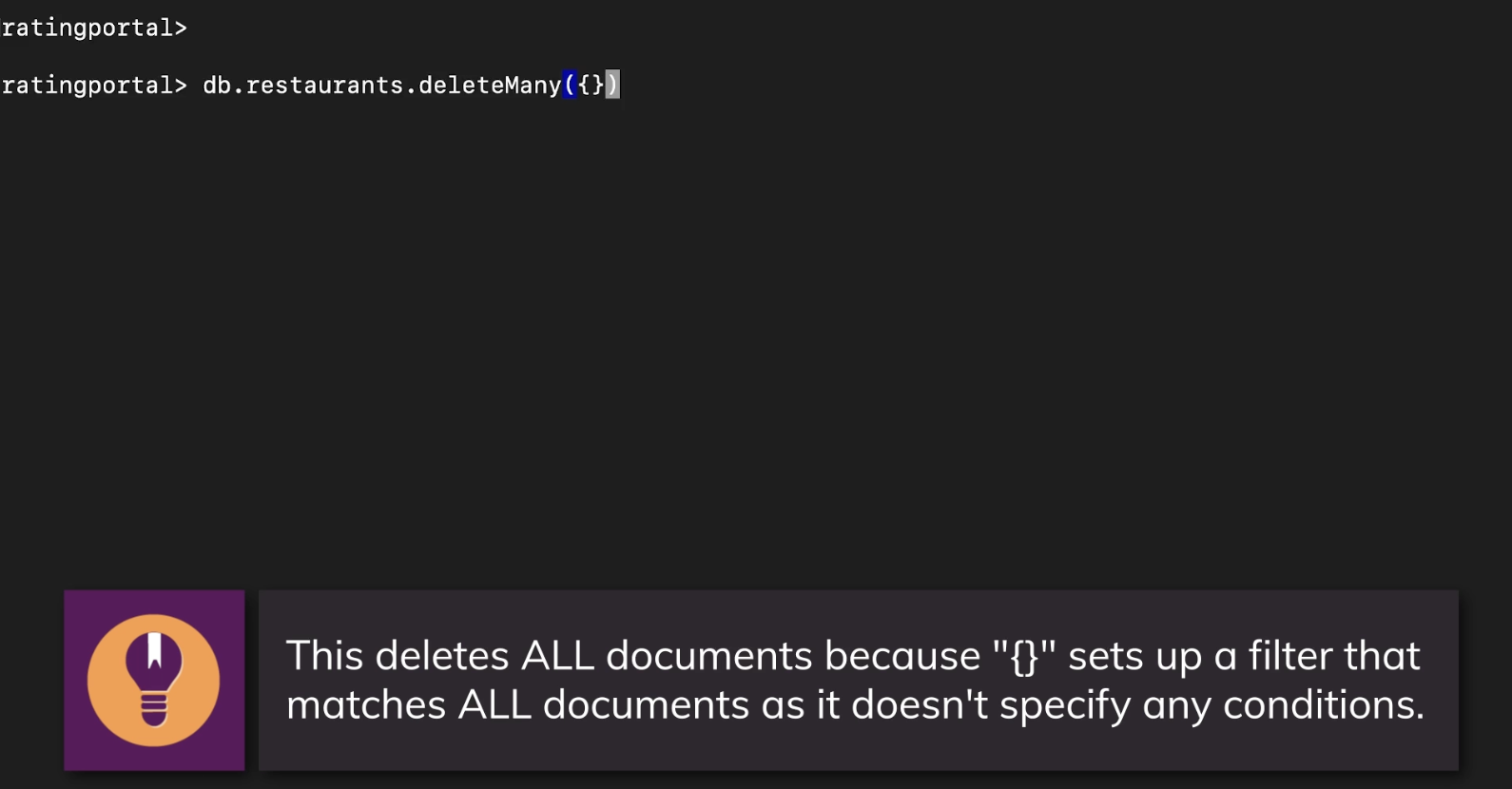
Since we are gonna store our data, we need to delete the data and start everything from scratch!
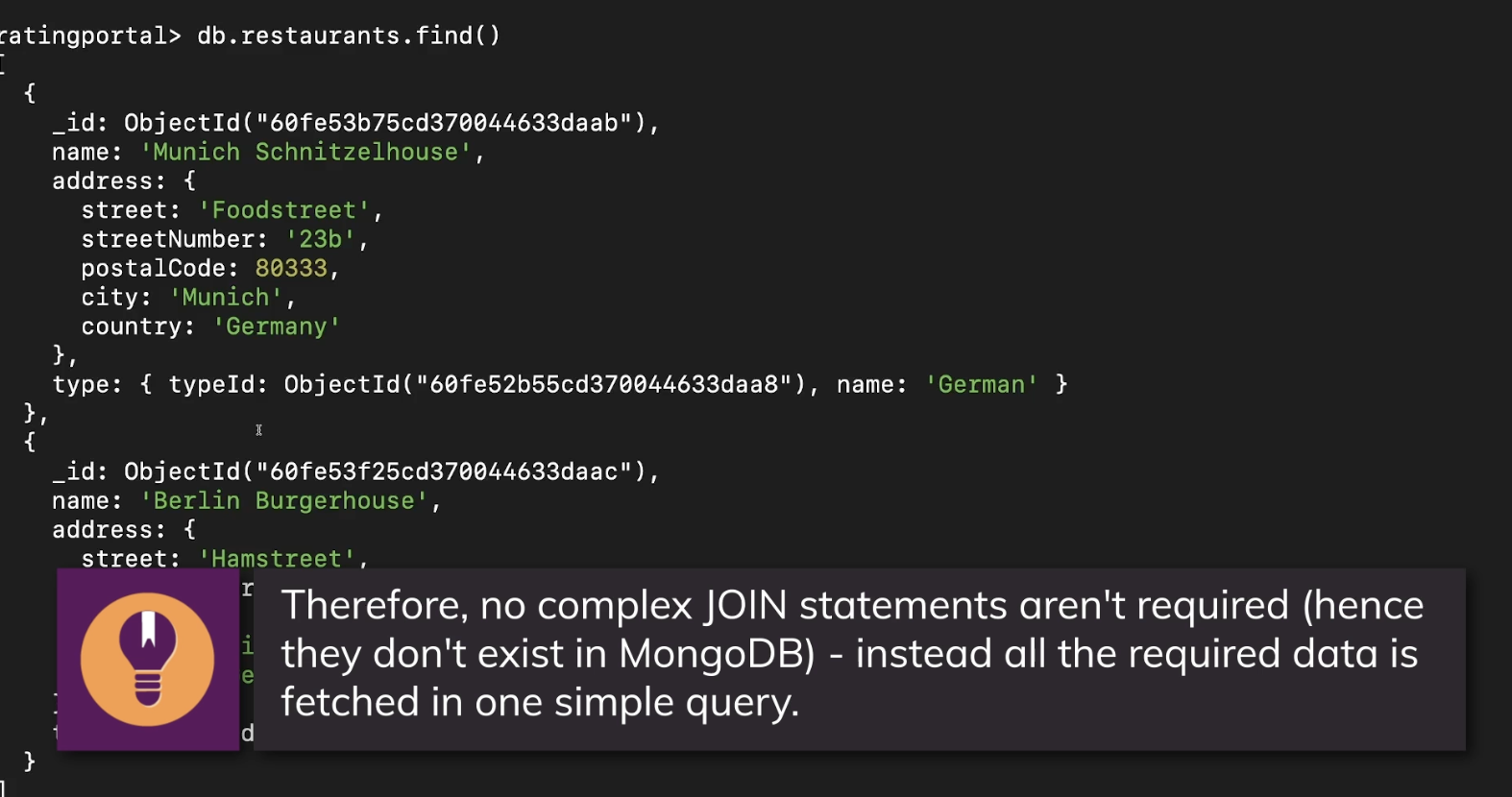
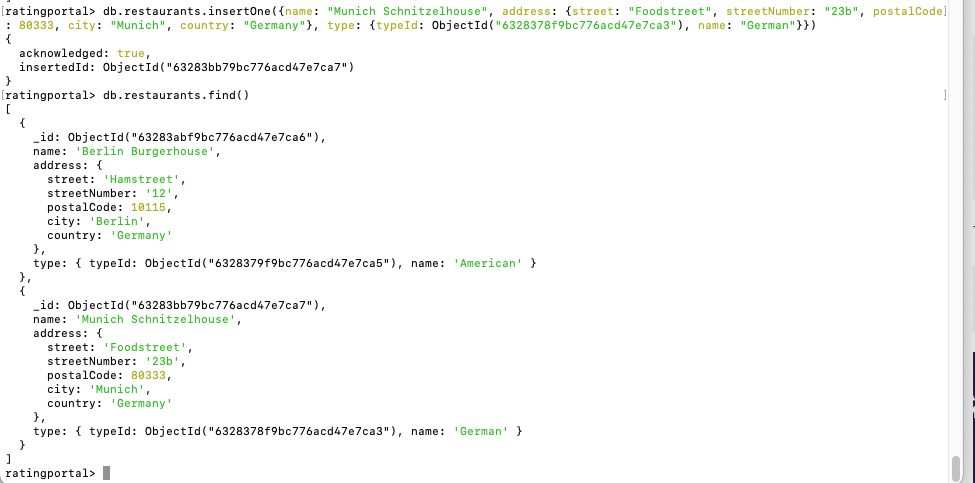
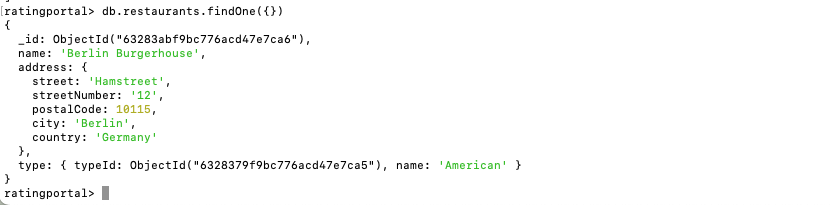
findOne only brings one data which is first.
mongoDB - Date()
Returns a date either as a string or as a Date object.
- Date() returns the current date as a string in mongosh.
- new Date() returns the current date as a Date object. mongosh wraps the Date object with the ISODate helper. The ISODate is in UTC.
You can specify a particular date by passing an ISO-8601 date string with a year within the inclusive range 0 through 9999 to the new Date() constructor or the ISODate() function. These functions accept the following formats:
- new Date("<YYYY-mm-dd>") returns the ISODate with the specified date.
- new Date("<YYYY-mm-ddTHH:MM:ss>") specifies the datetime in the client's local timezone and returns the ISODate with the specified datetime in UTC.
- new Date("<YYYY-mm-ddTHH:MM:ssZ>") specifies the datetime in UTC and returns the ISODate with the specified datetime in UTC.
- new Date(<integer>) specifies the datetime as milliseconds since the UNIX epoch (Jan 1, 1970), and returns the resulting ISODate instance.
Examples
If no document with _id equal to 1 exists in the products collection, the following operation inserts a document with the field dateAdded set to the current date:
db.products.updateOne(
{ _id: 1 },
{
$set: { item: "apple" },
$setOnInsert: { dateAdded: new Date() }
},
{ upsert: true }
)
Return Date as a String
To return the date as a string, use the Date() method, as in the following example:
var myDateString = Date();
Return Date as Date Object
mongosh wraps objects of Date type with the ISODate helper; however, the objects remain of type Date.
The following example uses new Date() to return Date object with the specified UTC datetime.
var myDate = new Date("2016-05-18T16:00:00Z");
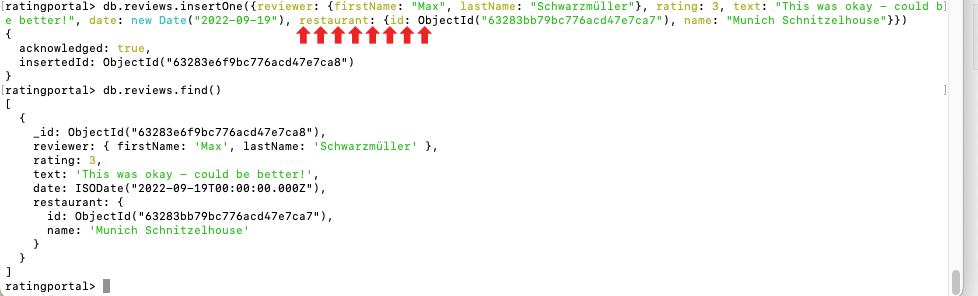
So here we can see that when we connect each database data, we just input the data here so we can connect each other.
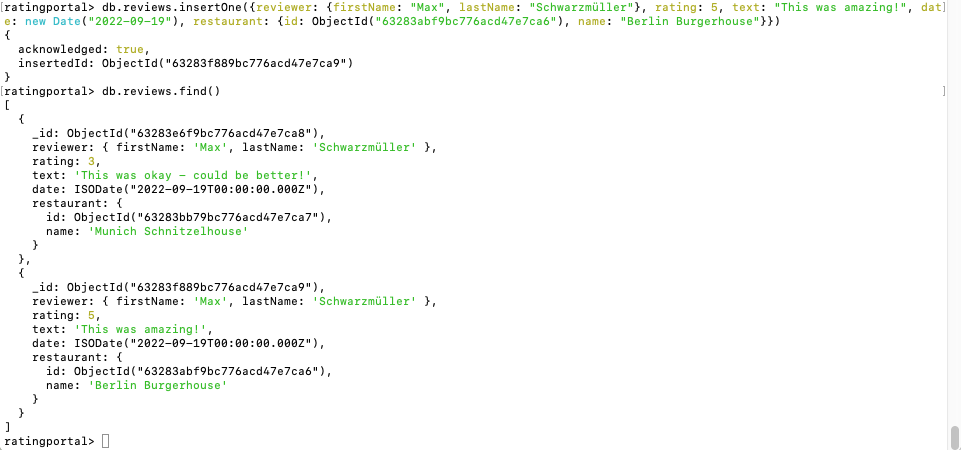
Comparison Query Operators
Comparison operators return data based on value comparisons.
NOTE
For details on a specific operator, including syntax and examples, click on the link to the operator's reference page.For comparison of different BSON type values, see the specified BSON comparison order.
Matches values that are equal to a specified value.
|
|
Matches values that are greater than a specified value.
|
|
Matches values that are greater than or equal to a specified value.
|
|
Matches any of the values specified in an array.
|
|
Matches values that are less than a specified value.
|
|
Matches values that are less than or equal to a specified value.
|
|
Matches all values that are not equal to a specified value.
|
|
Matches none of the values specified in an array.
|
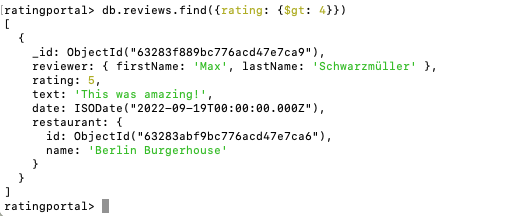
Sources
https://www.mongodb.com/docs/manual/reference/method/db.collection.updateMany/
db.collection.updateMany() — MongoDB Manual
Docs Home → MongoDB Manual db.collection.updateMany(filter, update, options)mongosh MethodThis page documents a mongosh method. This is not the documentation for a language-specific driver, such as Node.js.For MongoDB API drivers, refer to the language-s
www.mongodb.com
https://www.mongodb.com/docs/manual/reference/method/db.collection.deleteMany/
db.collection.deleteMany() — MongoDB Manual
Docs Home → MongoDB Manual db.collection.deleteMany()mongosh MethodThis page documents a mongosh method. This is not the documentation for a language-specific driver, such as Node.js.For MongoDB API drivers, refer to the language-specific MongoDB driver
www.mongodb.com
https://www.mongodb.com/docs/manual/reference/method/Date/
Date() — MongoDB Manual
Docs Home → MongoDB Manual Date()Returns a date either as a string or as a Date object.Date() returns the current date as a string in mongosh.new Date() returns the current date as a Date object. mongosh wraps the Date object with the ISODate helper. The
www.mongodb.com
https://www.mongodb.com/docs/manual/reference/operator/query-comparison/
Comparison Query Operators — MongoDB Manual
Docs Home → MongoDB Manual Comparison operators return data based on value comparisons.For details on a specific operator, including syntax and examples, click on the link to the operator's reference page.For comparison of different BSON type values, see
www.mongodb.com