In react, we try to use 'Ref' instead of using 'id'.
import React, { useRef } from "react";
import "./draggable.css";
export default function Draggable(children, handleRef, onMove) {
const dragRef = useRef(null)
return (
<div ref={dragRef} className="draggable" style={{transform: `translate(${0}px, ${0}px)`}}>
{children}
</div>
)
}
In this code, we use 'useRef' to make use of 'id' in regular code.
Element.getBoundingClientRect()
getBoundingClientRect()
Return value
The returned value is a DOMRect object which is the smallest rectangle which contains the entire element, including its padding and border-width. The left, top, right, bottom, x, y, width, and height properties describe the position and size of the overall rectangle in pixels. Properties other than width and height are relative to the top-left of the viewport.
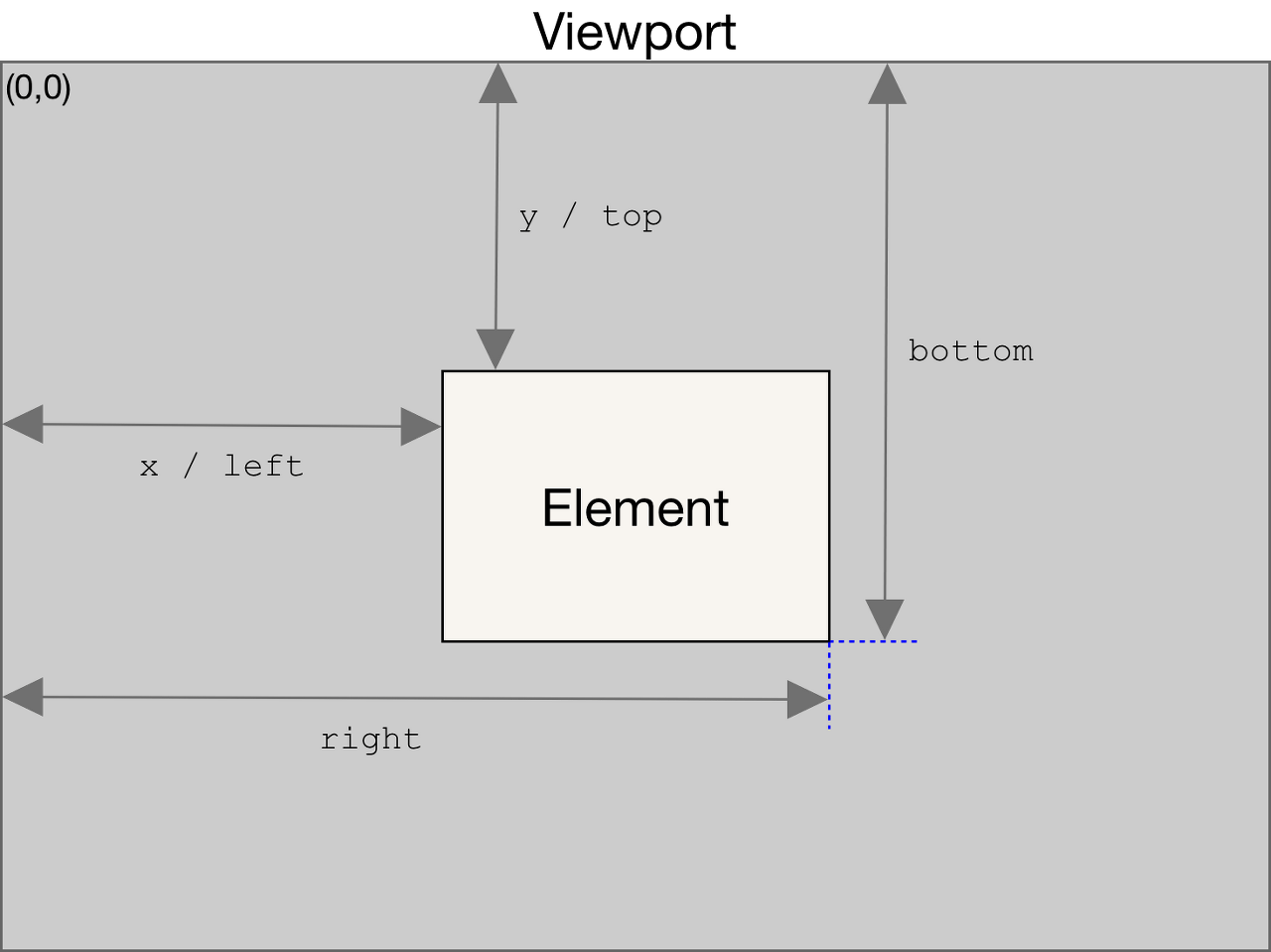
The width and height properties of the DOMRect object returned by the method include the padding and border-width, not only the content width/height. In the standard box model, this would be equal to the width or height property of the element + padding + border-width. But if box-sizing: border-box is set for the element this would be directly equal to its width or height.
The returned value can be thought of as the union of the rectangles returned by getClientRects() for the element, i.e., the CSS border-boxes associated with the element.
Empty border-boxes are completely ignored. If all the element's border-boxes are empty, then a rectangle is returned with a width and height of zero and where the top and left are the top-left of the border-box for the first CSS box (in content order) for the element.
The amount of scrolling that has been done of the viewport area (or any other scrollable element) is taken into account when computing the bounding rectangle. This means that the rectangle's boundary edges (top, right, bottom, left) change their values every time the scrolling position changes (because their values are relative to the viewport and not absolute).
If you need the bounding rectangle relative to the top-left corner of the document, just add the current scrolling position to the top and left properties (these can be obtained using window.scrollY and window.scrollX) to get a bounding rectangle which is independent from the current scrolling position.
So the logic is that we can get the current position of the cursor using 'getBoundingClientRect()'
We will subtract that current value from the first position to compare.
so everytime when our mouse is down at some point we will get the current value of position and then remove all events since we don't need anymore. (kinda resetting) and put it as initial value so we can compare next time also.
useCallback()
removeEventListener()
useEffect()
Here we used 'useEffect' for a clean-up.
throttle
debounce
If here we don't use debounce, then every time when we move the memo, it logs on console the position, so we set the interval of action.
Why we do publish?
-install npm i -D @babel/cli @babel/preset-react
-write codes in package.json to publish (scripts)
"publish": "rm -rf dist && mkdir dist && babel src/lib -d dist --copy-files"
-babel setting
"babel": {
"presets": ["@babel/preset-react"]
},
-basic info (remove private option) + should version up
"name": "@olivia/sticker-memo",
"version": "1.0.0",
"module": "dist/Draggable.js",
"main": "dist/Draggable.js",
-set gitignore again(added two lines to use 'dist')
/public
/src
-npm login (and then put info)
npm login
-publish (if it's first publish then put '--access public')
npm publish --access public
-now we can use this as package!
npm install @olivia-yj/sticker-memo
*but of course, we shouldn't forget that make a basic app.js, index.css file when we wanna use this package.
And we should give props also!
And now we can use this package to make our project!
Install mob X (store), sass, uuid (to modify or remove the specific item of memo)
Why here we used 'useMemo' is to remember debouncing.
If we use some function every 'onChange' then it will reoccur too many times
ResizeObserver
The ResizeObserver interface reports changes to the dimensions of an Element's content or border box, or the bounding box of an SVGElement.
Resize Observer는 설정한 요소의 크기 변화를 관찰하며,
크기 변화를 제어할 경우 발생할 수 있는 무한 콜백 루프나 순환 종속성(Circular dependency) 등의 다양한 문제 없이 사용할 수 있습니다.
Sources
https://developer.mozilla.org/en-US/docs/Web/API/Element/getBoundingClientRect
Element.getBoundingClientRect() - Web APIs | MDN
The Element.getBoundingClientRect() method returns a DOMRect object providing information about the size of an element and its position relative to the viewport.
developer.mozilla.org
https://developer.mozilla.org/en-US/docs/Web/API/ResizeObserver
ResizeObserver - Web APIs | MDN
The ResizeObserver interface reports changes to the dimensions of an Element's content or border box, or the bounding box of an SVGElement.
developer.mozilla.org
Resize Observer - 요소의 크기 변화 관찰
Resize Observer는 요소(Element)의 크기를 관찰하며, 요소의 크기가 변화할 때 실행할 최적화 콜백(callback)을 제공할 수 있습니다. 활용도를 높이기 위한 폴리필(polyfill) 사용법도 같이 알아봅니다.
heropy.blog