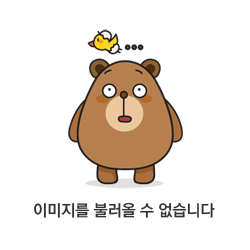
The goals
๐ช๐ปMore about Numbers
โ๐ปWorking with the Number & Math Objects
๐๐ปMore about Strings & Template Literals
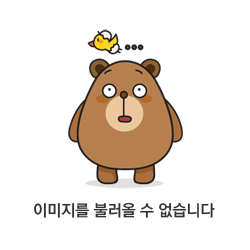
Javascript internally works with the binary system as we learned
Therefore Javascript converts this number to the binary system for doing the calculation and then basically converts it back to give us an output that makes sense to us humans because we rather work with the decimal system than with the binary system.
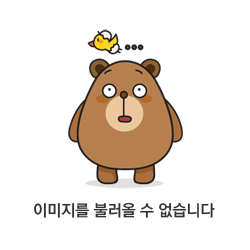
0.2 is equal to 1/5 in decimal system
0.4 is equal to 2/5 in decimal system
but in binary system, it is totally different number
So this is how Javascript performs the calculation and tries to show us the number we can understand
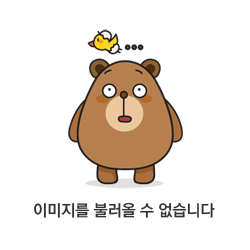
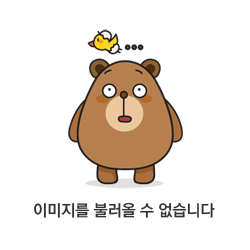
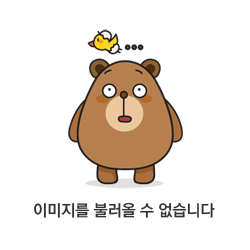
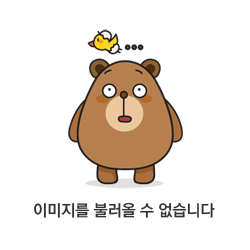
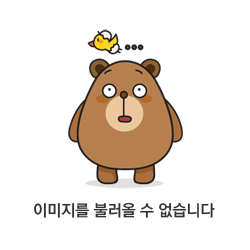
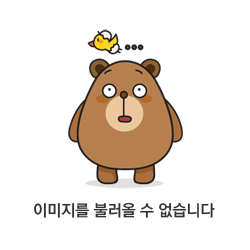
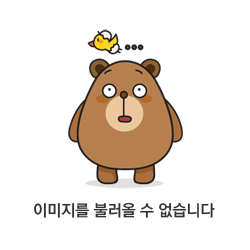
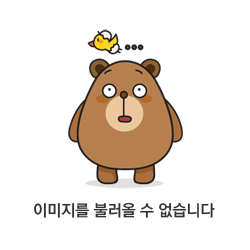
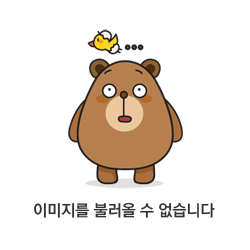
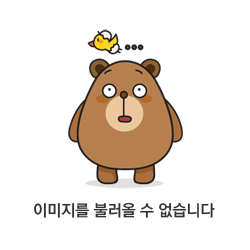
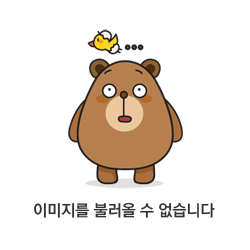
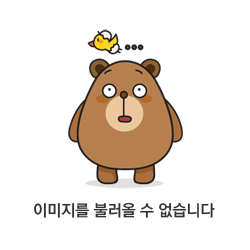
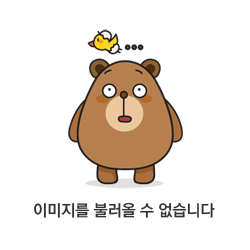
๊ทธ๋์ ์ฐ์ฐ์์ ์ ์ค์ฐจ๋๋ฌธ์ ์๋ฒฝํ ์ผ์นํ๋ ๊ฐ์ด ๋์ค์ง ์์์ ๋ฑ์์์ ์๋ฌ๊ฐ ๋ฐ์ํ๊ฒ ๋๋ ๊ฒ
ํด๊ฒฐ๋ฐฉ๋ฒ
1. ์ ํํ ๊ณ์ฐํ๋ ค๋ฉด ์ ์๋ก ํ๋ค (ํนํ๋ ์ ํํด์ผ ํ๋ ๋์ด๋ ์ฝ์ธ์ ๊ฒฝ์ฐ)
e.g. 5.1๋ฌ๋ฌ -> 5100์ผํธ
2. ๋ฐ์ฌ๋ฆผ ๋ฌธ๋ฒ์ ํ์ฉํ๋ค
e.g. precision = Math.pow(10, precision)
Math.ceil(num * precision) / precision
3. double ์๋ฃํ
์ ์ด์ ์ซ์๋ฅผ ๋ฉ๋ชจ๋ฆฌ์ ์ ์ฅํ ๋ ์ซ์ 1๊ฐ๋น 64์นธ์ ์ฌ์ฉํ๋๋ก ์ง์ ํจ
-> ๋จ์ : ๋ฉ๋ชจ๋ฆฌ ์ฉ๋ 2๋ฐฐ ํ์
์ด๋ฐ ์ ์ ์ ์๊ณ ์์ผ๋ฉด overflow, Not a Number, infinityํ์ ์ดํด์ ๋์์ด ๋จ!
BigInt()
BigInt๋ ์ซ์๊ฐ์ ์์ ์ ์ผ๋ก ๋ํ๋ผ ์ ์๋ ์ต๋์น๋ณด๋ค ํฐ ์ ์๋ฅผ ํํํ ์ ์๋ ์๋ก์ด ์์๊ฐ์ด๋ค. BigInt๊ฐ์ ์ ์ ๋ฆฌํฐ๋ด์ ๋ค์ n์ ๋ถ์ด๊ฑฐ๋ (10n) BigIntํจ์๋ฅผ ํธ์ถํด ์์ฑํ ์ ์๋ค.
BigInt is a primitive wrapper object used to represent and manipulate primitive bigint values โ which are too large to be represented by the number primitive.
A BigInt value, also sometimes just called a BigInt, is a bigint primitive, created by appending n to the end of an integer literal, or by calling the BigInt() function (without the new operator) and giving it an integer value or string value.
Math.random()
Math.floor()
Tagged Template
It can be useful when we have a scenario where we conveniently want to create some output, could be a string but could be something totally different as well, based on some string input.
What is Tagged Template Literals?
Tagged template literals were introduced in the ES6 version of JavaScript. Iโm safely assuming that most of you are hopefully aware of template strings, where you can interpolate dynamic content inside a string.
let greetings = "Hi";
let name = "Techsith";
let age = 35;
let statement = `${greetings} my name is ${name} and I am ${age}`;
The code sample above will render โHi my name is Techsith and I am 35โ as expected. In this template string, โ my name is โ and โ and I am โ are static content and greetings, name, and age are dynamic content. There are use cases where you want to take the user provided template string and perform some transformation to the dynamic content based on some logic. Tagged template literals let you extract all the dynamic values, which are called tags, and segregate them from the static content. This gives us the ability to transform these tags and interpolate it with the static content to derive a new string.
let greetings = "Hi";
let name = "Techsith";
let age = 35;
function transform(static, ...tags) {
console.log(static); // ["", " my name is ", " and I am ", ""]
console.log(tags); //["Hi", "Techsith", 35]
}
transform`${greetings} my name is ${name} and I am ${age}`;
In the above example, weโve created a function called transform. It takes two arguments: static array that holds all the static content and tags array that holds all the tags (dynamic content). When you run this function, it prints the following values, which are segregated based on content.
static => [โโ, โ my name is โ, โ and I am โ, โโ]
tags => [โHiโ, โTechsithโ, 35]
By interpolating static array with tags array, you can reconstruct the original string. When you dissect the output, youโll get empty strings if the tags are placed at the beginning or at the end of the string, respectively.
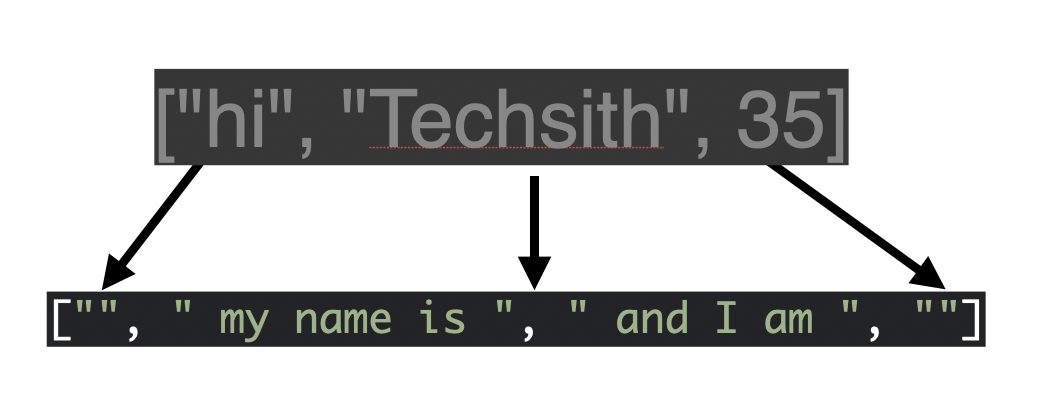
Letโs transform the name tag value Techsith to Hemil and reconstruct the string.
let greetings = "Hi";
let name = "Techsith";
let age = 35;
function transform(static, ...tags) {
let index = tags.findIndex((el) => el === "Techsith");
tags[index] = "Hemil";
let str = static[0];
for (i = 0; i < tags.length; i++) {
str += tags[i] + static[i + 1];
}
return str;
}
let newString = transform`${greetings} my name is ${name} and I am ${age}`;
console.log(newString); //"Hi my name is Hemil and I am 35"
RegEx
์ ๊ทํํ์ regular expression์ ์ผ์ ํ ํจํด์ ๊ฐ์ง ๋ฌธ์์ด์ ์งํฉ์ ํํํ๊ธฐ ์ํด ์ฌ์ฉํ๋ ํ์ ์ธ์ด (formal language)๋ค. ์ ๊ทํํ์์ ์๋ฐ์คํฌ๋ฆฝํธ์ ๊ณ ์ ๋ฌธ๋ฒ์ด ์๋๋ฉฐ, ๋๋ถ๋ถ์ ํ๋ก๊ทธ๋๋ฐ ์ธ์ด์ ์ฝ๋ ์๋ํฐ์ ๋ด์ฅ๋์ด ์๋ค. ์๋ฐ์คํฌ๋ฆฝํธ๋ ํ perl์ ์ ๊ท ํํ์ ๋ฌธ๋ฒ์ ES3๋ถํฐ ๋์ ํ๋ค.
์ ๊ทํํ์์ ๋ฌธ์์ด์ ๋์์ผ๋ก ํจํด ๋งค์นญ ๊ธฐ๋ฅ์ ์ ๊ณตํ๋ค. ํจํด ๋งค์นญ ๊ธฐ๋ฅ์ด๋ ํน์ ํจํด๊ณผ ์ผ์นํ๋ ๋ฌธ์์ด์ ๊ฒ์ํ๊ฑฐ๋ ์ถ์ถ ๋๋ ์นํํ ์ ์๋ ๊ธฐ๋ฅ์ ๋งํ๋ค.
Sources
https://www.youtube.com/watch?v=-GsrYvZoAdA&ab_channel=%EC%BD%94%EB%94%A9%EC%95%A0%ED%94%8C
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/BigInt
BigInt - JavaScript | MDN
BigInt is a primitive wrapper object used to represent and manipulate primitive bigint values โ which are too large to be represented by the number primitive.
developer.mozilla.org
https://patelhemil.medium.com/magic-of-tagged-templates-literals-in-javascript-e0e2379b1ffc
Magic of Tagged Templates Literals in JavaScript?
Once in a while you come across a JavaScript syntax that looks strange in nature. However, thatโs not quite true, letโs phrase itโฆ
patelhemil.medium.com
'JavaScript - The Complete Guide 2022' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
Section 18: Network Requests - Sending Http Requests via JavaScript (0) | 2022.11.03 |
---|---|
Section 17: Asynchronous Code - Code That "Takes a Bit Longer" (0) | 2022.11.02 |
Section 15: Functions - Advanced Concepts - That's More (0) | 2022.11.01 |
Section 14: Events - Beyond "click" Listeners (0) | 2022.11.01 |
Section 13: Advanced DOM Interactions - Beyond Querying & Inserting (0) | 2022.10.31 |