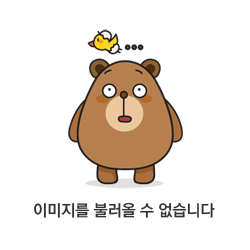
The goals
💪🏻What & Why?
✌🏻Request & Response Handling With Express
👍🏻Parsing & Storing User Input
Node Package Manager (NPM)
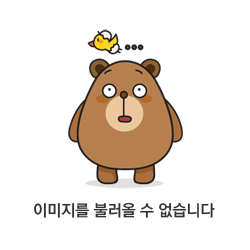
Try Express
const express = require('express');
const app = express();
app.get('/currenttime', function(req, res) {
res.send('<h1>' + new Date().toISOString() + '</h1>');
}); // localhost:3000/currenttime
app.get('/', function(req, res) {
res.send('<h1>Hello World!</h1>');
}); // localhost:3000/
app.listen(3000)
Express.js req.body
The req.body property contains key-value pairs of data submitted in the request body. By default, it is undefined and is populated when you use a middleware called body-parsing such as express.urlencoded() or express.json().
e.g.
var express = require('express');
var app = express();
var PORT = 3000;
// For parsing application/json
// To use middleware
app.use(express.json());
// For parsing application/x-www-form-urlencoded
app.use(express.urlencoded({ extended: true }));
app.post('/profile', function (req, res) {
console.log(req.body);
res.send();
});
app.listen(PORT, function(err){
if (err) console.log(err);
console.log("Server listening on PORT", PORT);
});
writeFileSync() / readFileSync()
The ‘fs’ module of Node.js implements the File I/O operation. Methods in the fs module can be synchronous as well as asynchronous. The Asynchronous function has a callback function as the last parameter which indicates the completion of the asynchronous function. Node.js developers prefer asynchronous methods over synchronous methods as asynchronous methods never block a program during its execution, whereas the later does. Blocking the main thread is malpractice in Node.js, thus synchronous functions should only be used for debugging or when no other options are available. The fs.writeFileSync() is a synchronous method. The fs.writeFileSync() creates a new file if the specified file does not exist. Also the ‘readline-sync’ module is used to enable user input at runtime.
Syntax:
fs.writeFileSync( file, data, options )
Parameters: This method accept three parameters as mentioned above and described below:
- file: It is a string, Buffer, URL or file description integer that denotes the path of the file where it has to be written. Using a file descriptor will make the it behave similar to fs.write() method.
- data: It is a string, Buffer, TypedArray or DataView that will be written to the file.
- options: It is an string or object that can be used to specify optional parameters that will affect the output. It has three optional parameter:
- encoding: It is a string which specifies the encoding of the file. The default value is ‘utf8’.
- mode: It is an integer which specifies the file mode. The default value is 0o666.
- flag: It is a string which specifies the flag used while writing to the file. The default value is ‘w’.
Below examples illustrate the fs.writeFileSync() method in Node.js:
Example 1:
// Node.js program to demonstrate the
// fs.writeFileSync() method
// Import the filesystem module
const fs = require('fs');
let data = "This is a file containing a collection"
+ " of programming languages.\n"
+ "1. C\n2. C++\n3. Python";
fs.writeFileSync("programming.txt", data);
console.log("File written successfully\n");
console.log("The written has the following contents:");
console.log(fs.readFileSync("programming.txt", "utf8"));
app.post('/store-user', function(req, res) {
const userName = req.body.username;
const filePath = path.join(__dirname, 'data', 'users.json');
const fileData = fs.readFileSync(filePath);
const existingUsers = JSON.parse(fileData);
existingUsers.push(userName);
fs.writeFileSync(filePath, JSON.stringify(existingUsers));
res.send('<h1>Username stored!</h1>');
});
path.join(__dirname)
The path.join() method is used to join a number of path-segments using the platform-specific delimiter to form a single path. The final path is normalized after the joining takes place. The path-segments are specified using comma-separated values.
Syntax:
path.join( [...paths] )
Parameters: This function accepts one parameter as mentioned above and described below:
- paths: It is a comma-separated sequence of paths that would be joined together to make the final path.
Return Value: It returns a string with the complete normalized path containing all the segments.
Below examples illustrate the path.join() method in Node.js:
Example 1:
- Node.js
// Node.js program to demonstrate the
// path.join() Method
// Import the path module
const path = require('path');
// Joining 2 path-segments
path1 = path.join("users/admin/files", "index.html");
console.log(path1)
// Joining 3 path-segments
path2 = path.join("users", "geeks/website", "index.html");
console.log(path2)
// Joining with zero-length paths
path3 = path.join("users", "", "", "index.html");
console.log(path3)
Output:
users\admin\files\index.html
users\geeks\website\index.html
users\index.html
app.post('/store-user', function(req, res) {
const userName = req.body.username;
const filePath = path.join(__dirname, 'data', 'users.json');
const fileData = fs.readFileSync(filePath);
const existingUsers = JSON.parse(fileData);
existingUsers.push(userName);
fs.writeFileSync(filePath, JSON.stringify(existingUsers));
res.send('<h1>Username stored!</h1>');
});
path.join vs path.resolve
there is a difference between the functions but the way you are using them in this case will result in the same outcome.
path.join returns a normalized path by merging two paths together. It can return an absolute path, but it doesn't necessarily always do so.
For instance:
path.join('app/libs/oauth', '/../ssl')
resolves to app/libs/ssl
path.resolve, on the other hand, will resolve to an absolute path.
For instance, when you run:
path.resolve('bar', '/foo');
The path returned will be /foo since that is the first absolute path that can be constructed.
However, if you run:
path.resolve('/bar/bae', '/foo', 'test');
The path returned will be /foo/test again because that is the first absolute path that can be formed from right to left.
If you don't provide a path that specifies the root directory then the paths given to the resolve function are appended to the current working directory. So if your working directory was /home/mark/project/:
path.resolve('test', 'directory', '../back');
resolves to
/home/mark/project/test/back
Using __dirname is the absolute path to the directory containing the source file. When you use path.resolve or path.join they will return the same result if you give the same path following __dirname. In such cases it's really just a matter of preference.
JSON (JavaScript Object Notation)
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language Standard ECMA-262 3rd Edition - December 1999. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
nodemon
npm install nodemon --save-dev
devDependencies
we will only use this modules while developing
Package.json - scripts
"start" : "nodemon app.js"
instead of npm run app.js
Sources
https://www.geeksforgeeks.org/express-js-req-body-property/
Express.js req.body Property - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
www.geeksforgeeks.org
https://www.json.org/json-en.html
JSON
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language Standard ECMA-262 3rd Edition
www.json.org
https://stackoverflow.com/questions/39110801/path-join-vs-path-resolve-with-dirname
path.join vs path.resolve with __dirname
Is there a difference when using both path.join and path.resolve with __dirname for resolving absolute path in Node.js? Should one of them be preferred when being used like that (absolute path
stackoverflow.com