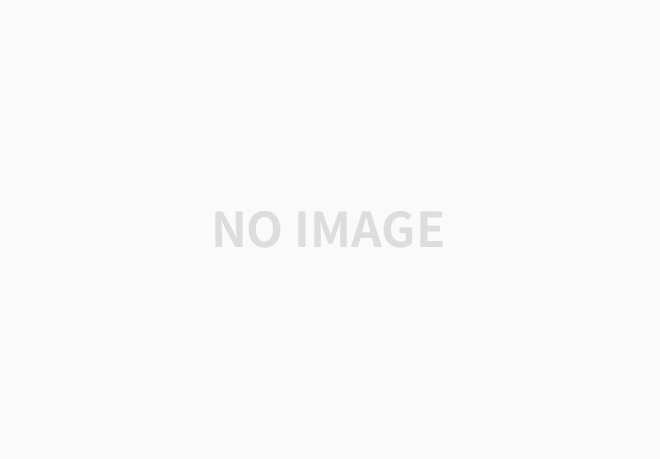
Global vs Local Components
In Vue.js, local components and global components refer to two different ways of defining and using components within a Vue application.
Local Components: Local components, also known as "scoped components," are components that are defined and registered within a specific Vue component. These components are only accessible and usable within the parent component and its child components. Local components provide encapsulation and reusability within a specific component or component hierarchy. They are typically defined using the components option within a Vue component.
Example of defining and using a local component:
// Parent component
const ParentComponent = {
components: {
LocalComponent: {
// Local component options
}
},
// ...
};
// Usage in template
<template>
<div>
<local-component></local-component>
</div>
</template>
In this example, LocalComponent is a local component defined within the ParentComponent using the components option. It can be used within the template of ParentComponent or any of its child components.
- Global Components: Global components, as the name suggests, are components that are registered globally and can be used across multiple Vue components within an application. Once registered globally, these components become available in the template of any Vue component without the need for explicit registration. Global components are typically registered using the Vue.component() method.
Example of defining and using a global component:
// Global component registration
Vue.component('GlobalComponent', {
// Global component options
});
// Usage in any template
<template>
<div>
<global-component></global-component>
</div>
</template>
In this example, GlobalComponent is a global component registered using Vue.component(). Once registered, it can be used in the template of any Vue component within the application without the need for additional registration steps.
Global components are useful for creating reusable components that can be shared and utilized across different parts of an application. However, it's important to use global components judiciously and consider the modular structure of the application to avoid potential naming conflicts or excessive global component registrations.
In summary, local components are defined and registered within a specific Vue component and are accessible only within that component and its children, while global components are registered globally and can be used in any Vue component without explicit registration.
Slots
In Vue.js, slots are a powerful feature that allows you to pass content from a parent component to its child component for rendering. Slots enable flexible and dynamic component composition by providing a way to inject content into a component template.
Here's how slots work:
Defining Slots in the Parent Component: In the template of a parent component, you can define one or more slots using the <slot> element. Each slot can have a name, allowing you to differentiate between multiple slots within the same component.
<template>
<div>
<slot></slot>
<slot name="footer"></slot>
</div>
</template>
In this example, the parent component defines two slots: a default slot represented by <slot></slot>, and a named slot with the name "footer" represented by <slot name="footer"></slot>.
Using Slots in the Child Component: In the template of a child component, you can use the <slot> element to indicate where the content from the parent component should be injected. You can also provide fallback content that will be displayed if no content is provided from the parent component.
<template>
<div>
<slot>
<!-- Fallback content if no content is provided -->
Default Content
</slot>
<footer>
<slot name="footer">
<!-- Fallback content for named slot if no content is provided -->
Default Footer
</slot>
</footer>
</div>
</template>
In this example, the child component uses <slot> to specify the insertion points for the content coming from the parent component. The fallback content will be displayed if no content is provided from the parent.
Passing Content to Slots: When using the parent component, you can pass content to the slots defined in the parent component by placing the desired content between the opening and closing tags of the parent component.
<parent-component>
<p>This content will be injected into the default slot.</p>
<template v-slot:footer>
<p>This content will be injected into the named 'footer' slot.</p>
</template>
</parent-component>
n this example, content is passed to the default slot and the named slot "footer" using the <template> element with the v-slot directive. The content defined within the <template> element will replace the respective slots in the parent component.
Slots provide a powerful way to create reusable components with flexible content injection.
In Vue.js, slots are a feature that allows you to define placeholders in a component's template where content can be inserted from the parent component. Slots provide a way to pass content and customize the rendering of a component without the need for explicit props passing.
Slots allow you to create reusable components that can accept different content or variations in their appearance and behavior. They provide a flexible and dynamic way to compose components and make them more adaptable to different use cases.
There are two types of slots in Vue.js:
Named Slots: Named slots allow you to define multiple placeholders within a component's template and assign specific content to each slot from the parent component. By using the <slot> element with a name attribute, you can define the location where the content will be inserted.
Example of a component with named slots:
<!-- Parent component -->
<template>
<div>
<child-component>
<template v-slot:header>
<!-- Content for the "header" slot -->
</template>
<template v-slot:body>
<!-- Content for the "body" slot -->
</template>
<template v-slot:footer>
<!-- Content for the "footer" slot -->
</template>
</child-component>
</div>
</template>
In this example, the parent component is using the <child-component> and providing content for different named slots (header, body, and footer). The child component's template defines the named slots using <slot> with matching names, allowing the provided content to be inserted at the appropriate locations.
Default Slot: The default slot is used when no explicit slot name is provided. It allows you to insert content into the component without specifying a specific named slot.
Example of a component with a default slot:
<!-- Parent component -->
<template>
<div>
<child-component>
<!-- Content for the default slot -->
</child-component>
</div>
</template>
In this example, the parent component is providing content directly between the opening and closing tags of the <child-component>. This content will be inserted into the default slot of the child component.
Slots provide a powerful way to create flexible and reusable components in Vue.js. They enable component composition and allow the parent component to customize and control the rendering of child components. By using slots effectively, you can create more versatile and adaptable Vue components.
Teleport
In Vue.js, the <teleport> component, also known as the <portal> component in some other frameworks, allows you to render a component's content in a different place in the DOM tree, outside of its current parent component. It provides a way to render content at a different location without breaking the component hierarchy or structure.
The <teleport> component is particularly useful when you need to render content in a different part of the DOM, such as moving a component's content to the body element or rendering it inside a specific container outside of the component's current context. It enables you to control the rendering position of a component's content while maintaining the component's logical structure.
To use <teleport>, you specify a target element or component using the to attribute, and then wrap the content that you want to teleport within the <teleport> tags.
Here's an example of using <teleport> in Vue.js:
<template>
<div>
<h1>Welcome to My App</h1>
<teleport to="body">
<!-- Content to be teleported -->
<MyComponent />
</teleport>
</div>
</template>
In this example, the content of the <teleport> component, which is the <MyComponent />, will be rendered in the <body> element instead of its original location within the component hierarchy. This allows you to render the component's content at a different place in the DOM, outside of its current parent component.
By using <teleport>, you have fine-grained control over where the content is rendered, allowing you to achieve various rendering scenarios. It can be helpful when dealing with z-index stacking contexts, modal dialogs, popovers, tooltips, and similar cases where you need to render content outside of the current component's context.
It's worth noting that <teleport> is available in Vue.js 3 and later versions. If you are using Vue.js 2, you can achieve similar functionality using third-party libraries like portal-vue.

Sources
https://webruden.tistory.com/923
Vue 슬롯(slot) 사용법 및 예제 (Understanding Slot in Vue.js)
Vue.js에서 컴포넌트를 활용해서 코드를 재사용함으로써 업무 효율성 및 생산성을 향상시킬 수 있습니다. 추가적으로 컴포넌트에 슬롯(Slots)을 활용하면 훨씬 재사용하기 용이하게 만들 수 있습
webruden.tistory.com