Section 12: JavaScript: Working with the DOM - Manipulating the running website
The goals
💪🏻Working with "the DOM" & Events
✌🏻Control Structures
👍🏻Examples!
Window & Document
Built-in Variables & Functions
Global "window" object - Holds information and functionality related to the active browser window / tab
e.g. current location, utility functions like alert()
The "document" object (window.document) - Holds information and functionality to the loaded website content
e.g. utility functions to access HTML elements
The DOM
Document Object Model
The data representation ("internal representation") of the parsed HTML code
The browser parses our HTML code and saves all elements as JavaScript objects
Our JavaScript code is able to interact with the DOM and extract data from it or manipulate it
Selecting DOM elements
"Drill into Elements"
document.body.children[0].firstChild
-> we have to know the DOM structure and if it changes, our code needs to change as well
"Query Elements"
document.getElementById('some-id');
document.querySelector('.some-class');
Selecting elements works like in CSS and hence no exact DOM structure knowledge is required
What's the Difference between DOM Node and Element?
The Document Object Model (DOM) is an interface that treats HTML or XML document as a tree structure, where each node is an object of the document. DOM also provides a set of methods to query the tree, alter the structure, style.
DOM also uses the term element: which is quite similar to a node. So, what's the difference between a DOM node and an element? Let's find out!
DOM Node
The key to understanding the difference between a node and an element is to understand what a node is.
From a higher viewpoint, a DOM document consists of a hierarchy of nodes. Each node can have a parent and/or children.
Let's look at the following HTML document:
<!DOCTYPE html>
<html>
<head>
<title>My Page</title>
</head>
<body>
<!-- Page Body -->
<h2>My Page</h2>
<p id="content">Thank you for visiting my web page!</p>
</body>
</html>
The document contains the following hierarchy of nodes:
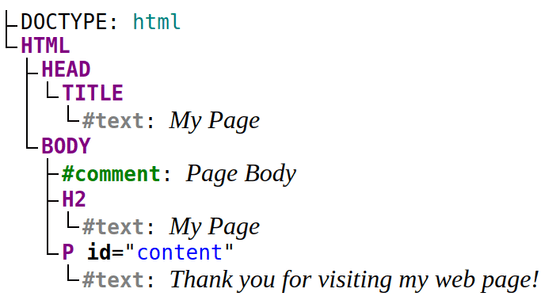
<html> is a node in the document tree. It has 2 children: <head> and <body> nodes.
<body> is also a node having 3 children: a comment <!-- Page Body -->, heading <h2>, and paragraph <p>. The parent of the <body> node is <html> node.
The tags in the HTML document represent a node, what's interesting is that regular text is also a node. The paragraph node <p> has 1 child: the text node "Thank you for visiting my web page!".
1.2 Node Types
How can you distinguish these different types of nodes? The answer lays in the DOM Node interface, particularly in the Node.nodeType property.
Node.nodeType can have one of the following values that represents the type of the node:
- Node.ELEMENT_NODE
- Node.ATTRIBUTE_NODE
- Node.TEXT_NODE
- Node.CDATA_SECTION_NODE
- Node.PROCESSING_INSTRUCTION_NODE
- Node.COMMENT_NODE
- Node.DOCUMENT_NODE
- Node.DOCUMENT_TYPE_NODE
- Node.DOCUMENT_FRAGMENT_NODE
- Node.NOTATION_NODE
The constants meaningfully indicate the node type: for example Node.ELEMENT_NODE represents an element node, Node.TEXT_NODE represents a text node, Node.DOCUMENT_NODE the document node, and so on.
For example, let's select the paragraph node, and look at its nodeType property:
const paragraph = document.querySelector('p');
paragraph.nodeType === Node.ELEMENT_NODE; // => true
As expected paragraph.nodeType has the value Node.ELEMENT_NODE, indicating that the paragraph is an element.
The paragraph also contains a text node:
const paragraph = document.querySelector('p');
const firstChild = paragraph.childNodes[0];
firstChild.nodeType === Node.TEXT_NODE; // => true
There's a node type that represents the entire document tree of nodes — Node.DOCUMENT_NODE:
document.nodeType === Node.DOCUMENT_NODE; // => true
DOM Element
After getting a good grasp of what a DOM node is, now is the time to differentiate the DOM node and element.
If you get well the node term, then the answer is obvious: an element is a node of a specific type — element (Node.ELEMENT_NODE). Along with types like document, comment, text, etc.
In simple words, an element is a node that's written using a tag in the HTML document. <html>, <head>, <title>, <body>, <h2>, <p> are all elements because they are represented by tags.
The document type, the comment, the text nodes aren't elements because they are not written with tags:
<!DOCTYPE html>
<html>
<body>
<!-- Page Body -->
<p>
Thank you for visiting my web page!
</p>
</body>
</html>
Node is constructor of a node, and HTMLElement is a constructor of an element in JavaScript DOM. A paragraph, being a node and also an element, is an instance of both Node and HTMLElement:
const paragraph = document.querySelector('p');
paragraph instanceof Node; // => true
paragraph instanceof HTMLElement; // => true
DOM properties: nodes and elements
Aside from differentiating nodes from elements, you need also to distinguish the DOM properties that contain specifically only nodes, or only elements.
The following properties of Node type evaluate to a node or a collection of nodes (NodeList):
node.parentNode; // Node or null
node.firstChild; // Node or null
node.lastChild; // Node or null
node.childNodes; // NodeList
However, the following properties are elements or collection of elements (HTMLCollection):
node.parentElement; // HTMLElement or null
node.children; // HTMLCollection
Consider the following paragraph element containing some text:
<p>
<b>Thank you</b> for visiting my web page!
</p>
Open the demo, then look at childNodes and children properties of the parapgraph node:
const paragraph = document.querySelector('p');
paragraph.childNodes; // NodeList: [HTMLElement, Text]
paragraph.children; // HTMLCollection: [HTMLElement]
paragraph.childNodes collection contains 2 nodes: the bold element <b>Thank you</b>, as well as the text node for visiting my web page!.
However, paragraph.children collection contains only 1 item: the bold element <b>Thank you</b>.
Because paragraph.children contains only elements, the text node wasn't included here because its type is text (Node.TEXT_NODE), and not an element (Node.ELEMENT_NODE).
Having both node.childNodes and node.children lets you choose the collection of children you'd like to access: all children nodes or only children being elements.
TextNode object
TextNode objects contain only text content without any HTML or XML markup. TextNode objects make it possible to insert texts into the document as nodes (appendChild, insertBefore).
The Difference Between an HTMLCollection and a NodeList
A NodeList and an HTMLcollection is very much the same thing.
Both are array-like collections (lists) of nodes (elements) extracted from a document. The nodes can be accessed by index numbers. The index starts at 0.
Both have a length property that returns the number of elements in the list (collection).
An HTMLCollection is a collection of document elements.
A NodeList is a collection of document nodes (element nodes, attribute nodes, and text nodes).
HTMLCollection items can be accessed by their name, id, or index number.
NodeList items can only be accessed by their index number.
An HTMLCollection is always a live collection. Example: If you add a <li> element to a list in the DOM, the list in the HTMLCollection will also change.
A NodeList is most often a static collection. Example: If you add a <li> element to a list in the DOM, the list in NodeList will not change.
The getElementsByClassName() and getElementsByTagName() methods return a live HTMLCollection.
The querySelectorAll() method returns a static NodeList.
The childNodes property returns a live NodeList.
Query Methods
- document.getElementById('some-id'): Select HTML element by id (id is unique so it would be only one element)
- document.querySelector('<some-css-selector>'): Select elements by CSS selector (ID selector, tag selector, class selector, combination selector), and bring the first item of it
- document.querySelectorAll('<some-css-selector>'): Bring all the elements have mentioned css selector
There are some other ways used rarely
- document.getElementsByClassName('some-css-class'): Choose all elements by class name
- document.getElementsByTagName('tag'): Choose all elements by tag name
Exercise!
addEventListener
The addEventListener() method of the EventTarget interface sets up a function that will be called whenever the specified event is delivered to the target.
Common targets are Element, or its children, Document, and Window, but the target may be any object that supports events (such as XMLHttpRequest).
syntax
addEventListener(type, listener);
addEventListener(type, listener, options);
addEventListener(type, listener, useCapture);
Sources
http://help.dottoro.com/ljkuedch.php
TextNode object JavaScript
Browser support: Represents a text as a node. Syntax: The base interface, through which you can add new functionalities to the TextNode object, is the Text interface. Possible members: Properties: baseURI Returns the base URL for the object. data Sets or r
help.dottoro.com
What's the Difference between DOM Node and Element?
What's the difference between a DOM node and an element? Let's find out!
dmitripavlutin.com