Section 10: Web Forms - Allowing Users To Enter Data
The goals
💪🏻What & Why?
✌🏻Exploring Different HTML Form Elements
👍🏻Building an Example Form
What are "Web Forms?"
Many websites are not just about presenting (showing) content => Instead, user input is often requested as well
e.g. Contact form, Checkout form, Login form, Comment form, etc....
There are special HTML elements for getting and handling user input
Key form elements
<input type ="...">
The "type" attribute controls which type of input will be shown (e.g. single line text input, number input, email address input...)
Every input requires its own lable
- "text" : A single-line text input field
- "email" : An input field optimized for email input (e.g. by showing an optimized keyboard on mobile phones)
- "number" : Optimized for number (with or without decimals) input
- "password" : A password input field where the entered characters are hidden
- "date" : An input field where the browser opens a date picker overlay
- "radio" : A radio button can be used to present multiple options where only one option can be selected simultaneously, they should have the same name so the user can pick only one option.
we use "value" attribute to choose what data we would like to send to the server. - "checkbox" : A checkbox button can be used to present a 'toggle' (yes / no) option to the visitor (e.g. "Agree to terms")
- "file" : A file picker allows the user to pick a file (e.g. for image upload)
- More input types are available, not all are supported in all browsers though (e.g. see MDN reference)
<textarea>
A multi- line text input field
<select>
A dropdown multiple-choice or multi-select input
<button>
A button to reset or sumbit the form
<main>
<form action="/" method="GET">
<section>
<h2>Your personal details</h2>
<ul>
<li>
<label for="username">Your name</label>
<input type="text" name="user-name" id="username" required placeholder="Max Schwarz" />
</li>
<li>
<label for="useremail">Your email address</label>
<input type="email" name="user-email" id="useremail" required />
</li>
<li>
<label for="userpassword">Your password</label>
<input type="password" name="user-password" id="userpassword" required minlength="7" />
</li>
</ul>
</section>
<section>
<h2>More details</h2>
<ul>
<li>
<label for="userage">Your age</label>
<input type="number" step="1" name="user-age" id="userage" required min="18" max="100" />
</li>
<li>
<label for="birthdate">Your birthdate</label>
<input type="date" id="birthdate" name="user-birthdate" required min="1921-01-01" max="2003-01-01" />
</li>
<li>
<label for="favorite-color">Your favorite color?</label>
<select id="favorite-color" name="favorite-color">
<option value="blue">My favorite color is blue</option>
<option value="black">Black</option>
<option value="red">Red</option>
</select>
</li>
</ul>
</section>
<section>
<label for="usermessage">Your message</label>
<textarea id="usermessage" name="user-message" required rows="8"></textarea>
</section>
<section>
<h2>How should we verify your account?</h2>
<ul>
<li class="form-control-inline">
<input
type="radio"
name="verify"
id="verify-text-message"
value="text-message"
required
/>
<label for="verify-text-message">Via text message (SMS)</label>
</li>
<li class="form-control-inline">
<input
type="radio"
name="verify"
id="verify-phone"
value="phone"
required
/>
<label for="verify-phone">Via a phone call</label>
</li>
<li class="form-control-inline">
<input
type="radio"
name="verify"
id="verify-email"
value="email"
required
/>
<label for="verify-email">Via an email</label>
</li>
</ul>
</section>
<section>
<h2>How should we contact you?</h2>
<ul>
<li class="form-control-inline">
<input
type="checkbox"
id="contact-email"
name="contact"
value="email"
/>
<label for="contact-email">Contact me via email</label>
</li>
<li class="form-control-inline">
<input
type="checkbox"
id="contact-phone"
name="contact"
value="phone"
/>
<label for="contact-phone">Contact me via phone</label>
</li>
</ul>
</section>
<hr />
<section>
<div class="form-control-inline">
<input type="checkbox" id="agree-terms" name="terms" />
<label for="agree-terms">Agree to terms?</label>
</div>
</section>
<section>
<button type="submit">Submit</button>
</section>
</form>
</main>
Inherit
The inherit CSS keyword causes the element to take the computed value of the property from its parent element. It can be applied to any CSS property, including the CSS shorthand property all.
For inherited properties, this reinforces the default behavior, and is only needed to override another rule.
Note: Inheritance is always from the parent element in the document tree, even when the parent element is not the containing block.
One Step Further
Now try to make another example from the scratch based on what I have learned!
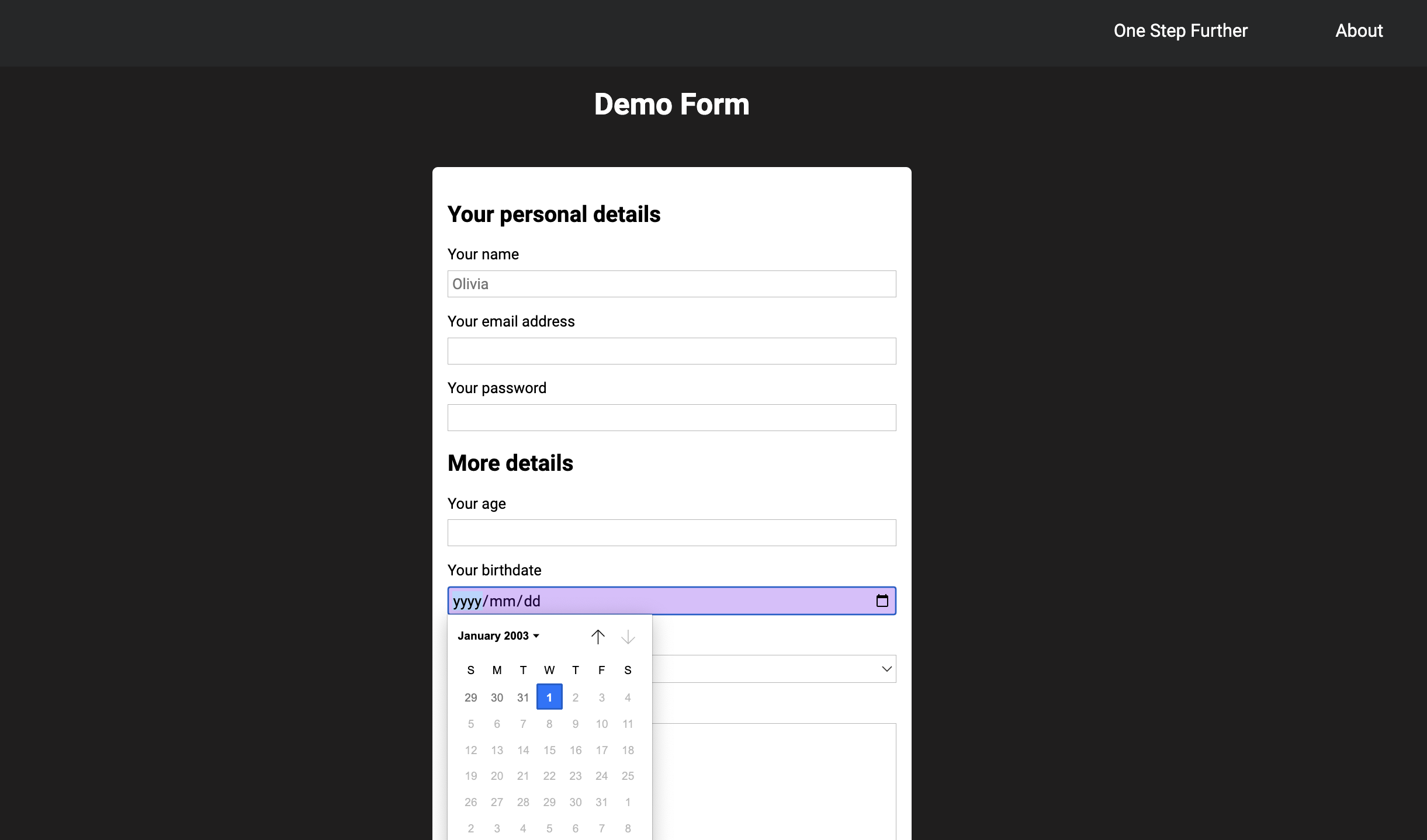
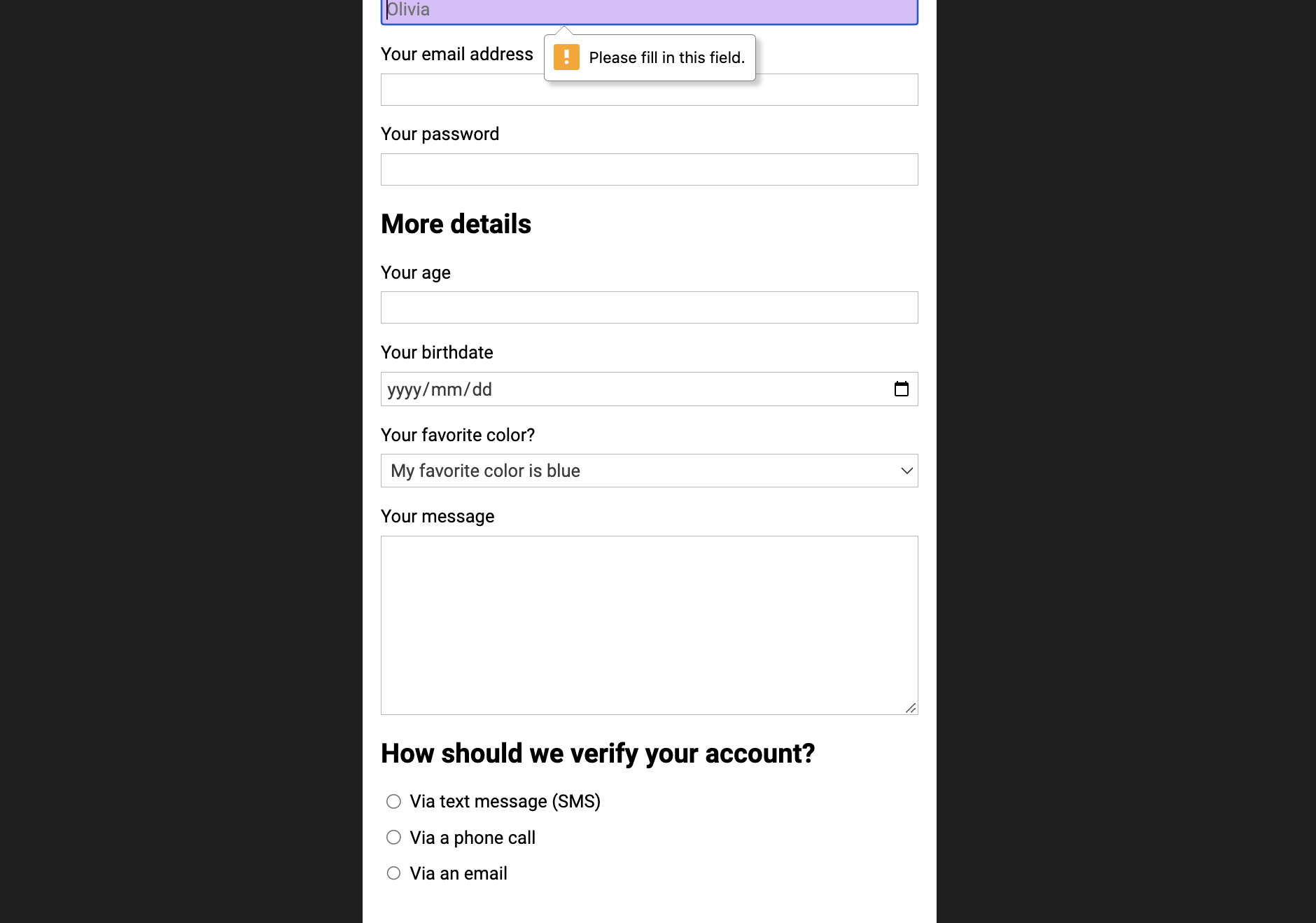
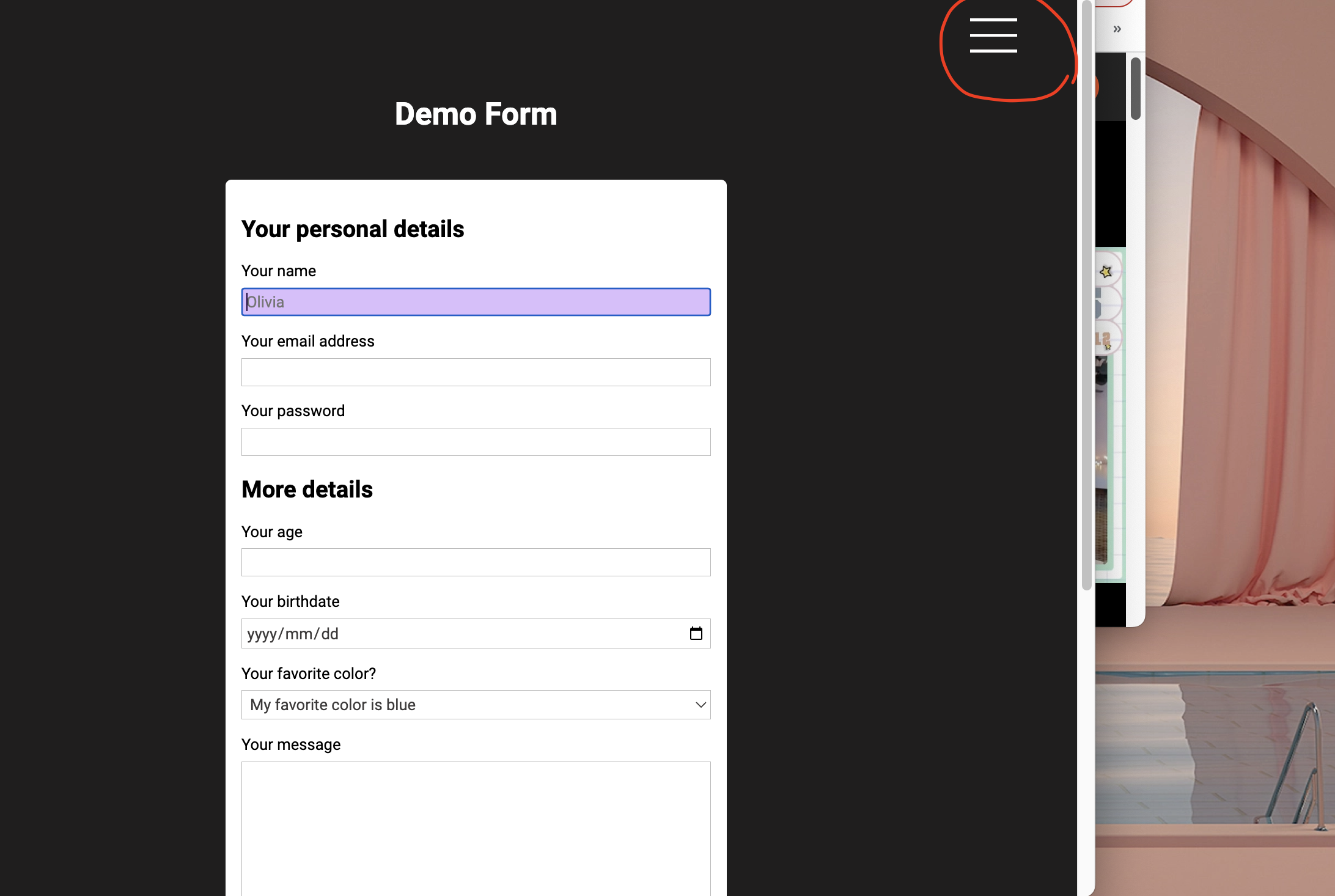
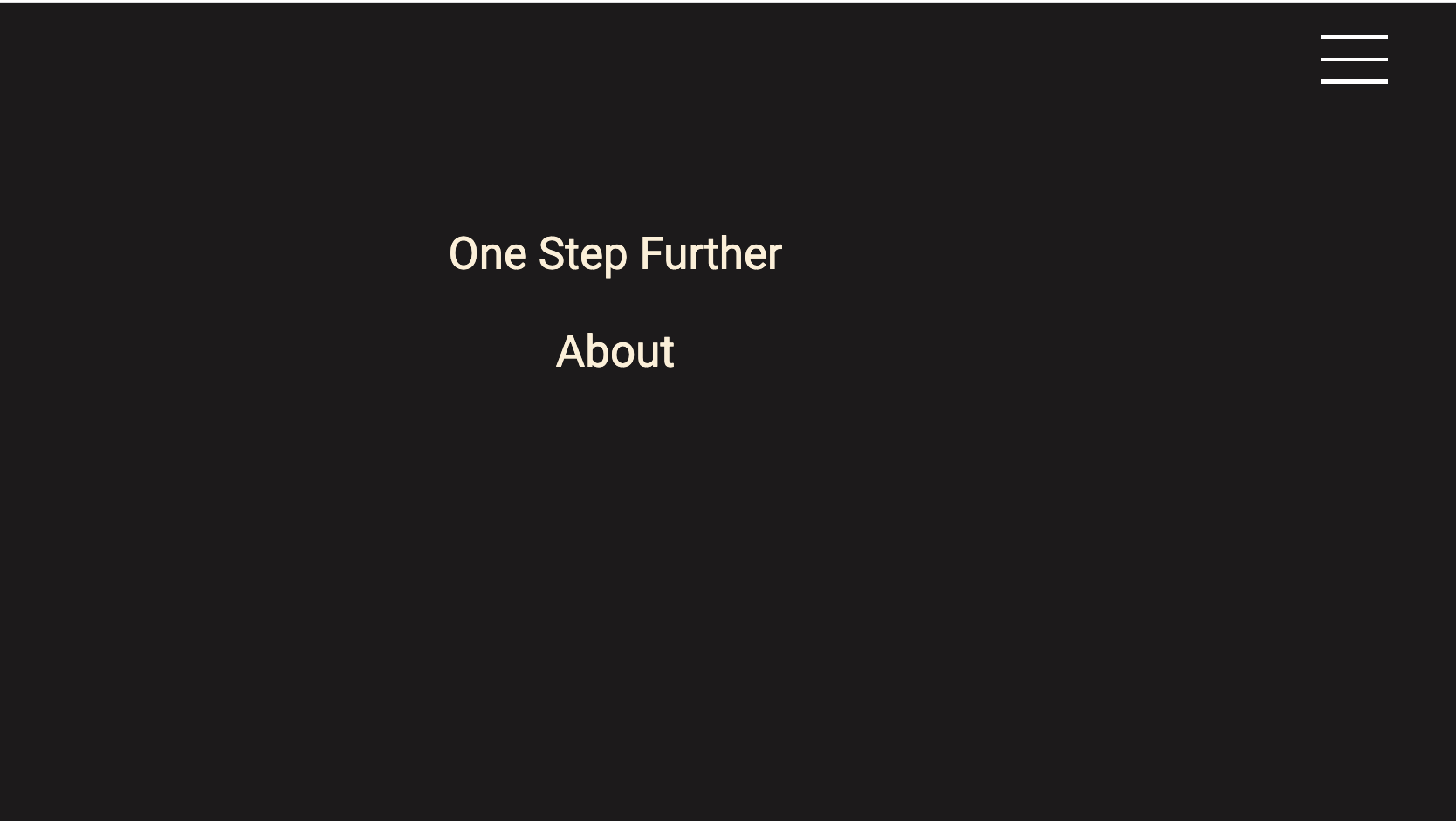
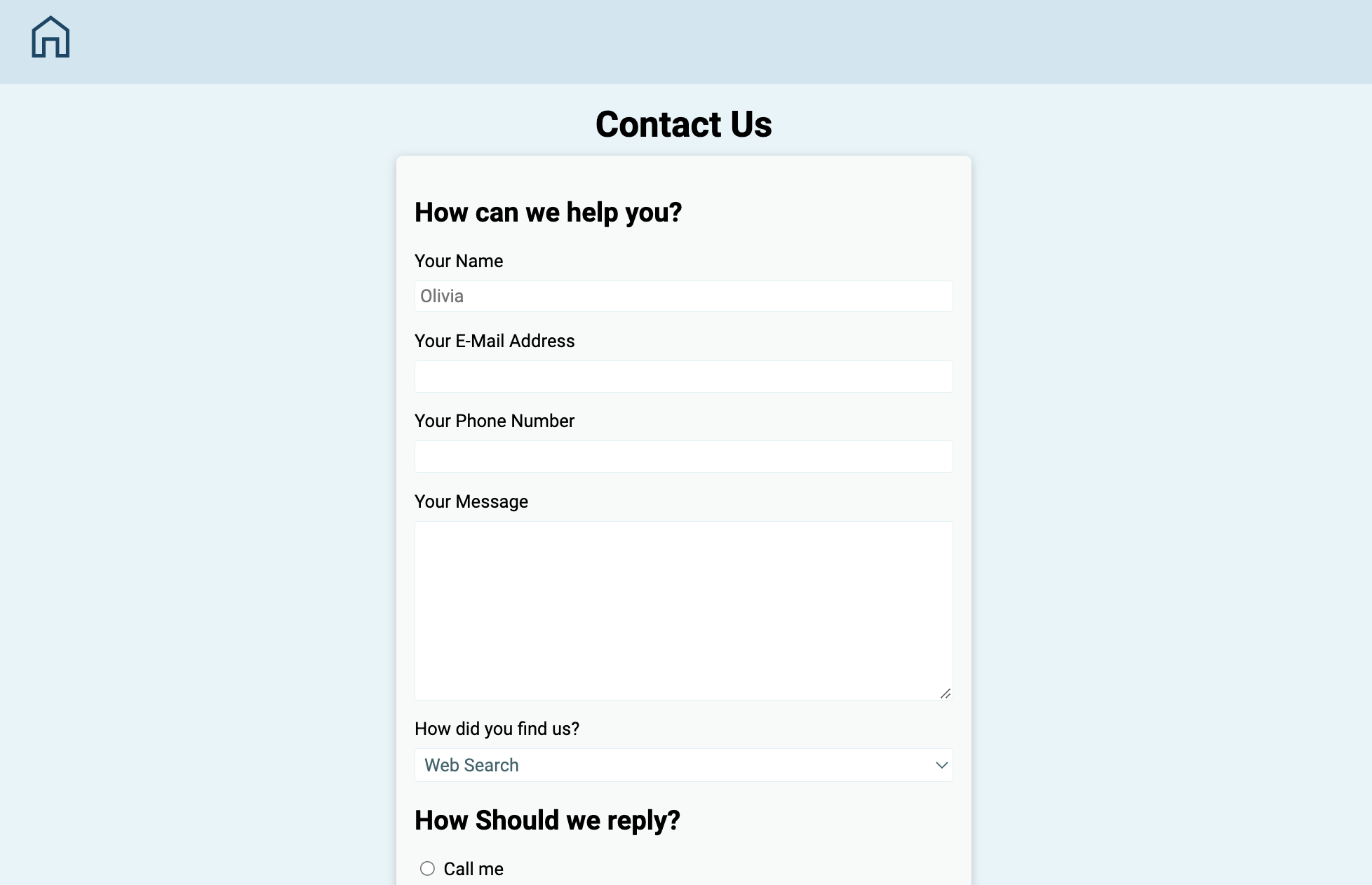
CSS tips
- Input element is automatically set as inline-block element, but we can overwrite with others.
- Before having form element, button didn't have any special role, but after we put form, it started to work as real submit button -> we can check it after clicking button and see the address of our page or check developer app and Network section.
- form has attributes such as action and type.
- form-method can be 'POST', 'GET', 'UPDATE' and 'DELETE'.
- The novalidate attribute is a boolean attribute. When present, it specifies that the form-data (input) should not be validated when submitted.
- We put 'required' as an option for each radio to make the user mandatorily choose one.
- We use lable to remember what the data means, because if we input some data then this placeholder would be gone, then we can't remember what it was about.
- If hovered item moves, then check margin and padding set on it. And if it is flex box item, then use space-between then it doesn't affect margin or padding.
- Justify-content we can use when it's flex item
- Flex-box only affects child itemsso if we wanna move buttons in section, then we should apply to section
or we can just use text-align cause buttons are inline-items